Course Title: Introduction to Java Programming: Build Your Foundation in Object-Oriented Programming
Curriculum:
- Java Basics: Learn the fundamental concepts of Java programming, including syntax, data types, and operators.
- Control Structures: Master control structures such as loops, conditionals, and switch statements.
- Object-Oriented Programming (OOP): Understand the principles of OOP, including classes, objects, inheritance, and polymorphism.
- Exception Handling: Learn how to handle errors and exceptions in Java programs.
- Java Collections Framework: Explore the Java Collections Framework, including lists, sets, and maps.
- File I/O: Work with files and streams to read and write data in Java.
- Introduction to Java APIs: Get familiar with essential Java APIs for building robust applications.
- Final Project: Develop a basic Java application that demonstrates your understanding of the concepts learned.
Long Description: Java is one of the most popular programming languages in the world, known for its versatility and wide range of applications. Our LearnPress course on Introduction to Java Programming is designed to help beginners build a strong foundation in object-oriented programming (OOP) using Java.
The course covers everything from basic syntax and control structures to more advanced topics like OOP principles and the Java Collections Framework. By the end of this course, you’ll have the skills needed to create basic Java applications and a solid understanding of OOP concepts. Learn more about why Java is a critical skill for software developers and how mastering it can open doors to numerous career opportunities. To further enhance your credentials, consider pursuing a cybersecurity certification to ensure your Java applications are secure and resilient.
2. Advanced Java Programming
Course Title: Advanced Java Programming: Master the Skills to Build Complex Applications
Curriculum:
- Advanced OOP Concepts: Delve deeper into advanced OOP concepts such as abstract classes, interfaces, and design patterns.
- Java Multithreading: Learn how to implement multithreading in Java to build responsive and high-performance applications.
- Java Networking: Explore Java networking concepts, including sockets, TCP/IP, and server-client communication.
- Java Database Connectivity (JDBC): Connect your Java applications to databases using JDBC.
- Java GUI Development: Build graphical user interfaces (GUIs) with JavaFX and Swing.
- Java Web Development: Learn the basics of web development with Java Servlets and JSP.
- Java Security: Implement security features in your Java applications, including encryption and authentication.
- Capstone Project: Develop a complex Java application that incorporates advanced features and demonstrates your mastery of Java.
Long Description: Take your Java programming skills to the next level with our LearnPress course on Advanced Java Programming. This course is designed for developers who have a basic understanding of Java and are looking to deepen their knowledge and build more complex applications.
You’ll explore advanced OOP concepts, multithreading, networking, and database connectivity, among other topics. The course also covers GUI development with JavaFX and Swing, as well as web development with Servlets and JSP. By the end of this course, you’ll be equipped to build sophisticated Java applications that are both functional and secure. Discover the benefits of advancing your Java programming skills and how it can lead to exciting career opportunities. To ensure your applications are secure, consider obtaining a cybersecurity certification to complement your Java expertise.
3. Java for Web Development
Course Title: Java for Web Development: Build Dynamic Websites and Applications with Java
Curriculum:
- Introduction to Web Development with Java: Understand the basics of web development and how Java fits into the picture.
- Java Servlets: Learn how to create dynamic web applications using Java Servlets.
- JavaServer Pages (JSP): Explore JSP and how it integrates with Servlets to build dynamic web pages.
- Model-View-Controller (MVC) Architecture: Implement the MVC architecture in Java web applications for better organization and maintainability.
- Database Integration: Connect your Java web applications to databases using JDBC and Hibernate.
- Session Management: Manage user sessions and state in Java web applications.
- Web Security: Implement security measures in your Java web applications, including authentication and encryption.
- Final Project: Build a dynamic web application using Java Servlets, JSP, and MVC architecture.
Long Description: Java is a powerful tool for web development, enabling developers to create dynamic and scalable web applications. Our LearnPress course on Java for Web Development is designed to teach you how to build websites and web applications using Java technologies like Servlets and JSP.
You’ll learn how to implement the Model-View-Controller (MVC) architecture, manage user sessions, and integrate databases into your web applications. The course also covers web security, ensuring your applications are protected from common threats. Explore how Java is used in web development and why it’s a valuable skill for aspiring web developers. To further secure your web applications, consider obtaining a cybersecurity certification to complement your web development skills.
4. Java for Android Development
Course Title: Java for Android Development: Create Powerful Mobile Applications with Java
Curriculum:
- Introduction to Android Development: Learn the basics of Android development and how Java is used to build Android apps.
- Android Studio Setup: Set up your development environment with Android Studio.
- UI Design with XML: Design user interfaces for Android apps using XML.
- Java for Android: Understand how Java is used to handle logic and functionality in Android apps.
- Activity Lifecycle: Learn about the Android activity lifecycle and how to manage app states.
- Data Storage: Implement data storage solutions in Android apps, including SharedPreferences and SQLite databases.
- Networking in Android: Learn how to implement networking features in Android apps, such as HTTP requests and RESTful APIs.
- Publishing Apps: Understand the process of publishing Android apps to the Google Play Store.
- Capstone Project: Develop a fully functional Android app using Java, showcasing your ability to create mobile applications.
Long Description: Java is the primary language used for Android development, making it essential for anyone looking to build mobile applications. Our LearnPress course on Java for Android Development is designed to teach you how to create powerful and engaging Android apps from scratch.
You’ll learn how to set up your development environment, design user interfaces, and handle app logic using Java. The course also covers data storage, networking, and the process of publishing apps to the Google Play Store. By the end of this course, you’ll have the skills needed to build and publish your own Android apps. Discover the advantages of using Java for Android development and how it can lead to a successful career in mobile app development. To ensure your mobile apps are secure, consider obtaining a cybersecurity certification to complement your Android development expertise.
5. Java Enterprise Edition (Java EE)
Course Title: Java Enterprise Edition (Java EE): Develop Robust Enterprise Applications
Curriculum:
- Introduction to Java EE: Learn the fundamentals of Java EE and its role in building enterprise-level applications.
- Java EE Components: Explore key components of Java EE, including Servlets, JSP, and Enterprise JavaBeans (EJB).
- Java Persistence API (JPA): Learn how to manage data persistence in Java EE applications using JPA.
- Java Messaging Service (JMS): Implement messaging features in your applications using JMS.
- JavaServer Faces (JSF): Build web interfaces for enterprise applications using JSF.
- Enterprise Application Architecture: Understand the architecture of enterprise applications and how Java EE supports it.
- Security in Java EE: Implement security features in your Java EE applications, including authentication and authorization.
- Final Project: Develop a robust enterprise application using Java EE technologies, demonstrating your ability to handle large-scale projects.
Long Description: Java Enterprise Edition (Java EE) is the backbone of many enterprise-level applications, providing the tools needed to build robust and scalable systems. Our LearnPress course on Java EE is designed to equip you with the skills needed to develop enterprise applications that meet the demands of large organizations.
You’ll learn about the key components of Java EE, including Servlets, JSP, EJB, and JPA, as well as how to implement messaging and web interfaces. The course also covers security, ensuring your enterprise applications are protected against common threats. Explore the benefits of using Java EE for enterprise development and how it can lead to a rewarding career in software development. To further enhance your skills, consider obtaining a cybersecurity certification to ensure your enterprise applications are secure and compliant.
6. Java Microservices Development
Course Title: Java Microservices Development: Build Scalable and Efficient Applications with Java
Curriculum:
- Introduction to Microservices: Learn the basics of microservices architecture and its advantages over monolithic applications.
- Java Frameworks for Microservices: Explore popular Java frameworks for building microservices, such as Spring Boot and MicroProfile.
- API Development: Learn how to develop RESTful APIs for microservices using Java.
- Service Communication: Implement inter-service communication in microservices using messaging protocols like Kafka and RabbitMQ.
- Data Management: Manage data across microservices using distributed databases and caching solutions.
- Security in Microservices: Implement security features in your microservices, including authentication, authorization, and encryption.
- Deployment and Scaling: Learn how to deploy and scale Java microservices using containerization and orchestration tools like Docker and Kubernetes.
- Final Project: Build a microservices-based application using Java, demonstrating your ability to create scalable and efficient systems.
Long Description: Microservices architecture is becoming increasingly popular for building scalable and maintainable applications. Our LearnPress course on Java Microservices Development is designed to teach you how to build efficient microservices using Java and modern frameworks like Spring Boot.
You’ll learn how to develop RESTful APIs, implement inter-service communication, and manage data across microservices. The course also covers security, deployment, and scaling, ensuring your microservices are both secure and performant. Discover the benefits of using Java for microservices development and how it can lead to opportunities in cloud computing and distributed systems. To ensure your microservices are secure, consider obtaining a cybersecurity certification to complement your microservices development expertise.
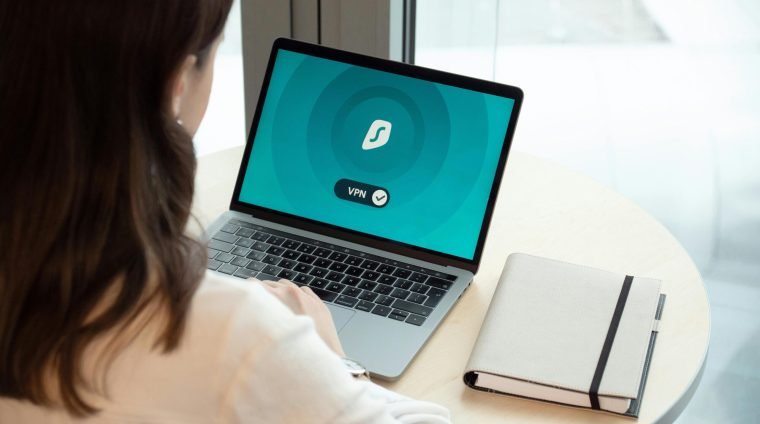