Introduction to React.js Development: Build Modern Web Applications
Introduction to React.js Development
Course Title: Introduction to React.js Development: Build Modern Web Applications
Curriculum:
- React.js Basics: Understand the fundamentals of React.js, including JSX, components, and props.
- Component Architecture: Learn how to create and manage React components and their states.
- Handling Events: Implement event handling and user interactions in React applications.
- State Management: Explore state management using React’s built-in hooks and Context API.
- Routing with React Router: Integrate React Router to handle navigation and routing.
- Data Fetching: Learn how to fetch and manage data from APIs within your React app.
- Styling React Components: Apply styling to components using CSS modules and styled-components.
- Final Project: Develop a complete React.js application that showcases your learning.
Long Description: Our LearnPress course on Introduction to React.js Development is perfect for beginners looking to build modern web applications. You’ll dive into React.js fundamentals, including JSX, components, and state management with hooks and Context API. This course also covers event handling, routing with React Router, and data fetching from APIs.
By the end of the course, you’ll have developed a fully functional React.js application, ready to enhance your web development skills. Discover the basics of React.js and its applications and how it can transform your web development approach. For securing your React apps, consider a cybersecurity certification.
2. Advanced React.js Development
Course Title: Advanced React.js Development: Master Complex React Applications
Curriculum:
- Advanced React Patterns: Explore advanced patterns like higher-order components and render props.
- Performance Optimization: Techniques for optimizing React application performance, including memoization and lazy loading.
- State Management Libraries: Learn to use state management libraries like Redux and MobX for complex applications.
- Custom Hooks: Create and use custom hooks to streamline your code.
- Testing React Applications: Implement testing strategies using tools like Jest and React Testing Library.
- Server-Side Rendering (SSR): Understand server-side rendering with Next.js for improved performance and SEO.
- API Integration: Advanced techniques for integrating and managing APIs in your React applications.
- Capstone Project: Build a complex React.js application incorporating advanced features and best practices.
Long Description: Our LearnPress course on Advanced React.js Development is designed for developers looking to enhance their skills in building sophisticated React applications. You’ll explore advanced patterns, performance optimization techniques, and state management libraries like Redux and MobX.
This course also covers custom hooks, testing strategies, and server-side rendering with Next.js. By the end, you’ll have built a complex React.js application that demonstrates your advanced skills. Learn advanced React.js techniques and how they can elevate your projects. For enhanced app security, consider a cybersecurity certification.
3. React.js for Front-End Development
Course Title: React.js for Front-End Development: Build Dynamic User Interfaces
Curriculum:
- Introduction to Front-End Development: Basics of front-end development and how React.js fits in.
- Building Dynamic UIs: Learn how to create responsive and interactive user interfaces with React.js.
- State Management with Hooks: Use React Hooks for managing component states effectively.
- Styling and Theming: Apply advanced styling techniques and theming for a polished UI.
- API Integration: Fetch and display data from APIs within your React.js application.
- Responsive Design: Implement responsive design principles to ensure your app works on various devices.
- Deployment: Learn how to deploy React.js applications to popular platforms like Vercel or Netlify.
- Final Project: Develop a dynamic front-end application that showcases your React.js skills.
Long Description: Our LearnPress course on React.js for Front-End Development focuses on building dynamic and interactive user interfaces. You’ll start with the basics of front-end development and dive into creating responsive UIs with React.js. The course includes advanced styling, API integration, and deployment techniques.
By the end of this course, you’ll have a dynamic front-end application ready for deployment, demonstrating your expertise in React.js. Explore front-end development with React.js and how it can improve your web design. Secure your application with a cybersecurity certification.
4. React.js and TypeScript Development
Course Title: React.js and TypeScript Development: Build Robust Applications
Curriculum:
- Introduction to TypeScript: Learn the fundamentals of TypeScript and its integration with React.js.
- Type Safety in React: Implement type safety and avoid common type-related errors in React applications.
- Advanced TypeScript Features: Explore advanced TypeScript features such as generics and type inference.
- Component Design with TypeScript: Design and build React components using TypeScript.
- State Management with TypeScript: Manage application state effectively using TypeScript.
- Testing with TypeScript: Learn testing strategies for TypeScript-based React applications.
- Deployment: Deploy your TypeScript and React.js application to cloud platforms.
- Capstone Project: Create a robust React.js application with TypeScript integration.
Long Description: Our LearnPress course on React.js and TypeScript Development provides a deep dive into building robust applications using TypeScript with React.js. You’ll learn TypeScript fundamentals, type safety in React, and advanced TypeScript features.
The course also covers component design, state management, and testing with TypeScript. By the end, you’ll have developed a solid React.js application with TypeScript, showcasing your advanced skills. Discover the benefits of using TypeScript with React.js and how it enhances your app development. For comprehensive app security, consider a cybersecurity certification.
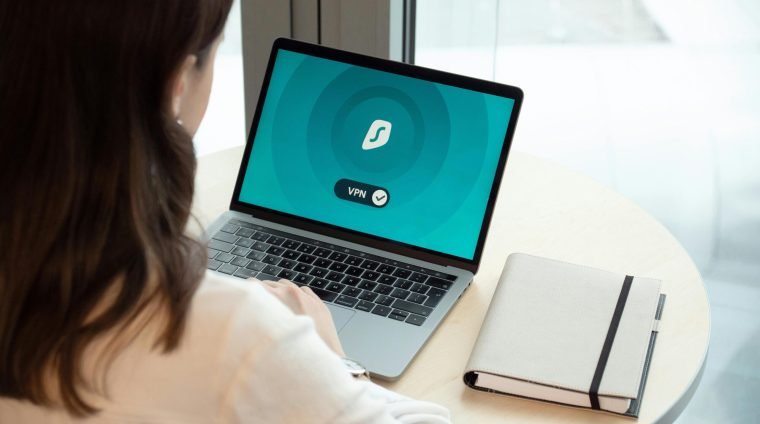