Introduction to C++ Programming: Learn the Fundamentals
Introduction to C++ Programming
Course Title: Introduction to C++ Programming: Learn the Fundamentals
Curriculum:
- C++ Basics: Understand the foundational concepts of C++ including syntax, data types, and operators.
- Control Structures: Explore control structures such as loops, conditionals, and functions.
- Object-Oriented Programming (OOP): Learn the principles of OOP including classes, objects, inheritance, and polymorphism.
- Memory Management: Understand dynamic memory allocation and management using pointers and references.
- Standard Template Library (STL): Get familiar with STL components like vectors, lists, and maps.
- Error Handling: Implement error handling using exception handling techniques.
- File I/O: Learn how to handle file input and output operations in C++.
- Final Project: Develop a complete C++ application to showcase your learning.
Long Description: Our LearnPress course on Introduction to C++ Programming is tailored for those new to programming or looking to solidify their C++ skills. You will start with C++ basics, including syntax, data types, and control structures. The course covers object-oriented programming principles, memory management, and the Standard Template Library (STL).
The curriculum also includes error handling and file I/O operations, culminating in a final project where you’ll develop a complete C++ application. Explore the fundamentals of C++ programming and how it can enhance your programming skills. For robust application security, consider a cybersecurity certification.
2. Advanced C++ Programming
Course Title: Advanced C++ Programming: Master Complex Concepts
Curriculum:
- Advanced OOP Concepts: Dive deeper into advanced object-oriented programming techniques including multiple inheritance and virtual functions.
- Templates and Generics: Learn to use C++ templates and generics for creating flexible and reusable code.
- Concurrency: Explore multithreading and concurrency in C++ for efficient program execution.
- Design Patterns: Study common design patterns used in C++ for solving recurring design problems.
- Performance Optimization: Techniques for optimizing C++ code for better performance.
- Memory Management: Advanced memory management techniques including smart pointers and custom allocators.
- Exception Safety: Implement advanced exception safety measures in your C++ applications.
- Capstone Project: Build a complex C++ application integrating advanced concepts and best practices.
Long Description: Our LearnPress course on Advanced C++ Programming is designed for experienced programmers looking to master complex C++ concepts. You’ll delve into advanced OOP techniques, templates, and concurrency. The course also covers design patterns, performance optimization, and advanced memory management.
By the end of the course, you’ll complete a complex C++ application demonstrating your advanced skills and understanding. Learn advanced C++ programming techniques and how they can elevate your development projects. For enhanced security, explore cybersecurity certification.
3. C++ Programming for Game Development
Course Title: C++ Programming for Game Development: Build Engaging Games
Curriculum:
- Game Development Basics: Understand the basics of game development and how C++ fits into the process.
- Game Loop and State Management: Learn to implement a game loop and manage different game states.
- Graphics and Rendering: Explore graphics programming and rendering techniques using libraries like SDL or SFML.
- Physics and Collision Detection: Implement basic physics simulations and collision detection for interactive gameplay.
- Audio Integration: Integrate audio into your games using libraries for sound effects and music.
- Optimizing Game Performance: Techniques for optimizing game performance and managing resources efficiently.
- Game Engine Basics: Introduction to using and customizing game engines for your C++ projects.
- Final Project: Create a complete game application demonstrating your skills in C++ game development.
Long Description: Our LearnPress course on C++ Programming for Game Development is designed for those interested in creating engaging games. You’ll start with the basics of game development and progress to implementing game loops, managing game states, and integrating graphics and audio.
The course also covers performance optimization and introduces game engines for more advanced projects. By the end, you’ll build a complete game application showcasing your C++ game development skills. Explore game development with C++ and its role in creating interactive experiences. For securing your game applications, consider a cybersecurity certification.
4. C++ Programming for Software Engineering
Course Title: C++ Programming for Software Engineering: Build Robust Software
Curriculum:
- Software Engineering Principles: Learn the core principles of software engineering and how to apply them using C++.
- Design and Architecture: Explore software design patterns and architecture principles for scalable and maintainable software.
- Testing and Debugging: Techniques for testing and debugging C++ applications effectively.
- Version Control: Implement version control practices using tools like Git.
- Documentation: Best practices for documenting C++ code and software projects.
- API Design: Learn how to design and implement APIs in C++ for modular and reusable code.
- Project Management: Understand project management methodologies and how they apply to software development.
- Capstone Project: Develop a robust software application that integrates best practices in software engineering.
Long Description: Our LearnPress course on C++ Programming for Software Engineering is ideal for those looking to build robust software systems. The course covers software engineering principles, design patterns, and architecture principles for creating scalable software.
You’ll also learn about testing, debugging, version control, and API design. The course culminates in a capstone project where you’ll develop a comprehensive software application. Discover software engineering with C++ and how it can improve your development skills. For added security, consider a cybersecurity certification.
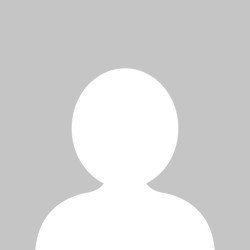
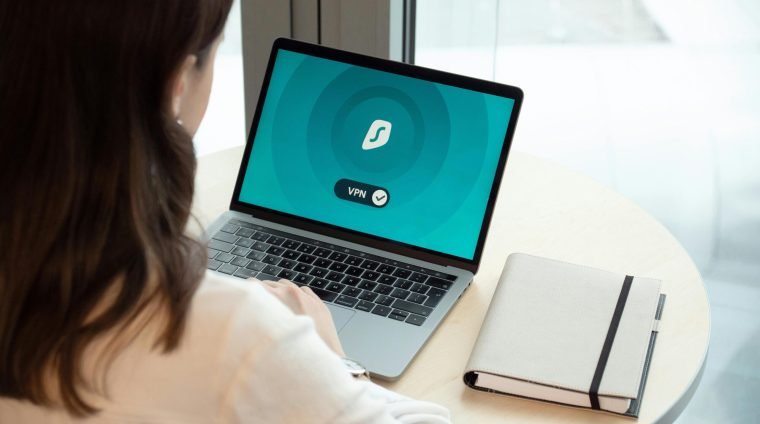